Below is a sample screen with a custom tab bar controller:
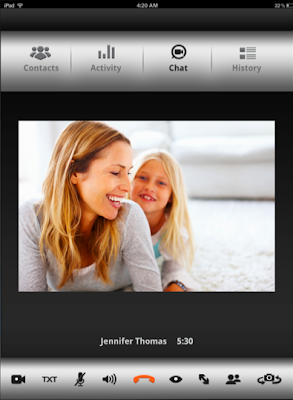
There is an example of a custom class for a UITabBarController with a backgroun image and 3 buttons with custom action & image.
This is the custom tab bar controller class can be look like:
// CustomTabBarController.h
#import
@interface CustomTabBarController : UITabBarController {
UIButton *settingsButton;
UIButton *infoButton;
UIButton *aboutUsButton;
}
@property (nonatomic, retain) UIButton *settingsButton;
@property (nonatomic, retain) UIButton *infoButton;
@property (nonatomic, retain) UIButton *aboutUsButton;
-(void) addCustomElements;
-(void) selectTab:(int)tabID;
@end
// CustomTabBarController.m
#import "CustomTabBarController.h"
@implementation CustomTabBarController
@synthesize settingsButton, infoButton, aboutUsButton;
- (void)viewDidAppear:(BOOL)animated {
[super viewDidAppear:animated];
}
-(void)viewDidLoad
{
[super viewDidLoad];
[self addCustomElements];
}
-(void)addCustomElements
{
// Background
UIImageView* bgView = [[[UIImageView alloc] initWithImage:
[UIImage imageNamed:@"tabBarBackground.png"]] autorelease];
bgView.frame = CGRectMake(0, 420, 320, 60);
[self.view addSubview:bgView];
// Initialise our two images
UIImage *btnImage = [UIImage imageNamed:@"settings.png"];
UIImage *btnImageSelected = [UIImage imageNamed:
@"settingsSelected.png"];//Setup the button
self.settingsButton = [UIButton buttonWithType:UIButtonTypeCustom];
// Set the frame (size and position) of the button)
settingsButton.frame = CGRectMake(10, 426, 100, 54);
// Set the image for the normal state of the button
[settingsButton setBackgroundImage:btnImage forState:
UIControlStateNormal];// Set the image for the highlighted state of the button
[settingsButton setBackgroundImage:btnImageSelected forState:
UIControlStateHighlighted];// Set the image for the selected state of the button
[settingsButton setBackgroundImage:btnImageSelected forState:
UIControlStateSelected];// Set the image for the disabled state of the button
[settingsButton setBackgroundImage:btnImageSelected forState:
UIControlStateDisabled];
[settingsButton setImage:btnImageSelected forState:
(UIControlStateHighlighted|UIControlStateSelected)];/* Assign the button a "tag" so when our "click" event is called
we know which button was pressed.*/[settingsButton setTag:101];
// Set this button as selected (we will select the others to false
as we only want Tab 1 to be selected initially).*/[settingsButton setSelected:true];
// Now we repeat the process for the other buttons
btnImage = [UIImage imageNamed:@"info.png"];
btnImageSelected = [UIImage imageNamed:@"infoSelected.png"];
self.infoButton = [UIButton buttonWithType:UIButtonTypeCustom];
infoButton.frame = CGRectMake(110, 426, 100, 54);
[infoButton setBackgroundImage:btnImage forState:
UIControlStateNormal];
[infoButton setBackgroundImage:btnImageSelected forState:
UIControlStateSelected];
[infoButton setBackgroundImage:btnImageSelected forState:
UIControlStateHighlighted];
[infoButton setImage:btnImageSelected forState:
(UIControlStateHighlighted|UIControlStateSelected)];
[infoButton setTag:102];
btnImage = [UIImage imageNamed:@"aboutUs.png"];
btnImageSelected = [UIImage imageNamed:@"aboutUsSelected.png"];
self.aboutUsButton = [UIButton buttonWithType:UIButtonTypeCustom];
aboutUsButton.frame = CGRectMake(210, 426, 100, 54);
[aboutUsButton setBackgroundImage:btnImage forState:
UIControlStateNormal];
[aboutUsButton setBackgroundImage:btnImageSelected forState:
UIControlStateSelected];
[aboutUsButton setBackgroundImage:btnImageSelected forState:
UIControlStateHighlighted];
[aboutUsButton setImage:btnImageSelected forState:
(UIControlStateHighlighted|UIControlStateSelected)];
[aboutUsButton setTag:103];
// Add my new buttons to the view
[self.view addSubview:settingsButton];
[self.view addSubview:infoButton];
[self.view addSubview:aboutUsButton];
/* Setup event handlers so that the buttonClicked method will
respond to the touch up inside event.*/
[settingsButton addTarget:self action:@selector(buttonClicked:)
forControlEvents:UIControlEventTouchUpInside];
[infoButton addTarget:self action:@selector(buttonClicked:)
forControlEvents:UIControlEventTouchUpInside];
[aboutUsButton addTarget:self action:@selector(buttonClicked:)
forControlEvents:UIControlEventTouchUpInside];
}
- (void)buttonClicked:(id)sender
{
int tagNum = [sender tag];
[self selectTab:tagNum];
}
- (void)selectTab:(int)tabID
{
switch(tabID)
{
case 101:
[settingsButton setSelected:true];
[infoButton setSelected:false];
[aboutUsButton setSelected:false];
break;
case 102:
[settingsButton setSelected:false];
[infoButton setSelected:true];
[aboutUsButton setSelected:false];
break;
case 103:
[settingsButton setSelected:false];
[infoButton setSelected:false];
[aboutUsButton setSelected:true];
break;
}
self.selectedIndex = tabID;
}
- (void)dealloc {
[settingsButton release];
[infoButton release];
[aboutUsButton release];
[super dealloc];
}
@end